|
1 |
| -# springboot--work-order-priority-queue |
2 |
| -A web service that accepts work orders and provides a prioritized list to work. |
| 1 | +# WORK ORDER PRIORITY QUEUE WEB SERVICES # |
| 2 | +- - - - - - - - |
| 3 | +    |
| 4 | +## Problem Statement ## |
| 5 | +_Create a web service that accepts work orders and provides a prioritized list to work._ |
| 6 | + |
| 7 | + |
| 8 | + |
| 9 | + |
| 10 | +Develop a Restful API for creating work order queue for Service Desk employee. The work order can be of four types `normal, priority, VIP, and management override`. Each type of request has it's own implementation for calculating rank. Different types of requests uses different formula to calculate the rank. The priority queue is sorted from highest ranked to lowest ranked ID. |
| 11 | + |
| 12 | +At the core the app uses a priority queue interface for creating work order queue. The priority queue is used to solve [many useful problems](https://en.wikipedia.org/wiki/Priority_queue#Applications). |
| 13 | + |
| 14 | +## GETTING STARTED ## |
| 15 | +This application is a written in java using [Spring Boot][1] and built using Maven. |
| 16 | + |
| 17 | +### Prerequisites ### |
| 18 | +* Git |
| 19 | +* JDK 8 or later |
| 20 | +* Maven 3.0 or later |
| 21 | + |
| 22 | +### Quick Start ### |
| 23 | +This would be really quick. To test the application, you just need to do these two things: |
| 24 | + |
| 25 | +1. Download the fat jar of this application from [bin](https://github.com/Govind-jha/springboot-work-order-priority-queue/tree/master/bin, "click here.") folder. Now to start the application server, open command prompt and run the downloaded `jar` file, |
| 26 | +``` |
| 27 | + java -jar workorder-1.0.0.one-jar |
| 28 | +``` |
| 29 | +2. Once the server gets started, download the postman collection form [postman](https://github.com/Govind-jha/springboot-work-order-priority-queue/tree/master/postman, "click here.") folder of the project. Import the collection in [Postman client](https://github.com/postmanlabs) and you will be all set to play around with the API. |
| 30 | + |
| 31 | +### Clone ### |
| 32 | +To get started you can simply clone this repository using git: |
| 33 | +``` |
| 34 | +git clone https://github.com/Govind-jha/springboot-work-order-priority-queue.git |
| 35 | +cd springboot-work-order-priority-queue |
| 36 | +``` |
| 37 | + |
| 38 | +### Build an executable JAR ### |
| 39 | +You can run the application from the command line using |
| 40 | +``` |
| 41 | + mvn spring-boot:run |
| 42 | +``` |
| 43 | +Or you can build a single executable JAR file that contains all the necessary dependencies, classes, and resources with |
| 44 | +``` |
| 45 | + mvn clean package |
| 46 | +``` |
| 47 | +Then you can run the JAR file with |
| 48 | +``` |
| 49 | + java -jar target/*.jar |
| 50 | +``` |
| 51 | +### Test ### |
| 52 | + |
| 53 | +>> Download the postman collection form [postman](https://github.com/Govind-jha/springboot-work-order-priority-queue/tree/master/postman, "click here.") folder. Import the collection in [Postman client](https://github.com/postmanlabs) for smoke testing. |
| 54 | +
|
| 55 | +You can test these Micro Services using any tool or language that allows you to make a HTTP request. For example, removing an order using [CURL](https://curl.haxx.se/): |
| 56 | +```sh |
| 57 | +curl -X DELETE \ |
| 58 | + http://localhost:8080/workorder/remove/20 \ |
| 59 | + -H 'Cache-Control: no-cache' |
| 60 | +``` |
| 61 | + |
| 62 | +Creating a work order in the queue using `require` module in [Node.js](https://github.com/nodejs), |
| 63 | + |
| 64 | +```js |
| 65 | +var request = require("request"); |
| 66 | + |
| 67 | +var options = { |
| 68 | + method: 'POST', |
| 69 | + url: 'http://localhost:8080/workorder/addOrder', |
| 70 | + headers: |
| 71 | + { |
| 72 | + 'Cache-Control': 'no-cache', |
| 73 | + 'Content-Type': 'application/json' |
| 74 | + }, |
| 75 | + body: { requesterId: 20, timeOfRequest: 5000000000 }, |
| 76 | + json: true |
| 77 | +}; |
| 78 | + |
| 79 | +request(options, function (error, response, body) { |
| 80 | + if (error) throw new Error(error); |
| 81 | + |
| 82 | + console.log(body); |
| 83 | +}); |
| 84 | +``` |
| 85 | + |
| 86 | +## PRE DEV ANALYSIS ## |
| 87 | + |
| 88 | +### Object Model ### |
| 89 | +``` |
| 90 | +PriorityQueue<WorkOrder>[ |
| 91 | + WorkOrder{ |
| 92 | + requestorID, |
| 93 | + requestType, |
| 94 | + requestTimestamp, |
| 95 | + requestDate |
| 96 | + }, ... |
| 97 | +] |
| 98 | +``` |
| 99 | +### Request Type Calculation ### |
| 100 | +There are 4 classes of IDs, normal, priority, VIP, and management override. You can determine the class of the ID using the following method, |
| 101 | + |
| 102 | + |
| 103 | +| Request Type | Condition | |
| 104 | +|-----------------|----------------------------------| |
| 105 | +| `NORMAL` | (requestorID % 3 != 0 && requestorID % 5 != 0) | |
| 106 | +| `PRIORITY` | requestorID % 3 == 0 | |
| 107 | +| `VIP` | requestorID % 5 == 0 | |
| 108 | +| `MANAGEMENT_OVERRIDE` | (requestorID % 3 == 0 && requestorID % 5 == 0) | |
| 109 | + |
| 110 | +### Rank Calculation Formula ### |
| 111 | +IDs must be sorted in the queue according to a formula that varies based on their class. The table below lists the formula to calculate the rank for different request types. |
| 112 | + |
| 113 | + |
| 114 | +| Request Type | Ranking Function | |
| 115 | +|--------------|----------------------------------| |
| 116 | +| `NORMAL` | secondsSpentInQueue | |
| 117 | +| `PRIORITY` | max( 3 , secondsSpentInQueue * log(secondsSpentInQueue) ) | |
| 118 | +| `VIP` | max( 4 , 2 * secondsSpentInQueue * log(secondsSpentInQueue) ) | |
| 119 | +| `MANAGEMENT_OVERRIDE` | max(NORMAL.maxPriority(), PRIORITY.maxPriority(), VIP.maxPriority()) + secondsSpentInQueue | |
| 120 | + |
| 121 | +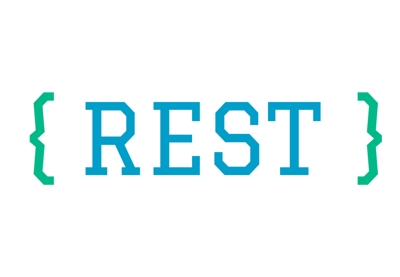 |
| 122 | + |
| 123 | +## API Contract ## |
| 124 | + |
| 125 | +The WorkOrder Api implements the requirement/rules described in the previous section. It is used to create a work order queue for service request desk employee. |
| 126 | + |
| 127 | +### Response Code And Headers ### |
| 128 | +If response status code is `204 NO CONTENT` or `205 RESET CONTENT`, the response will contain header `x-app-diagnostic`. HTTP status `205 RESET CONTENT` means server successfully processed the request, but is not returning any content. Unlike a `204 NO CONTENT` response, this response requires that the requester reset the document view (as the content might have changed). |
| 129 | + |
| 130 | +_Response header `x-app-diagnostic` will contain the cause of no content in the response body._ |
| 131 | + |
| 132 | +#### Response Codes |
| 133 | + |
| 134 | +|Status Code|Message| |
| 135 | +|-----------|-------| |
| 136 | +|`200` | Success | |
| 137 | +|`201` | Content Created| |
| 138 | +|`204` | No Content | |
| 139 | +|`205`| Reset Content| |
| 140 | +|`404`|Not Found| |
| 141 | +|`405`| Method Not Allowed| |
| 142 | +|`415`| Usupported Media Type| |
| 143 | + |
| 144 | +- Check the requests content type if you get HTTP Status `415`. |
| 145 | +- Check the requests Method(GET, POST, DELETE) if you get HTTP Status `405`. |
| 146 | + |
| 147 | +### API Operation ### |
| 148 | +Resource components can be used in conjunction with identifiers to retrieve the metadata for that identifier. |
| 149 | + |
| 150 | +| Base Resource | Description | |
| 151 | +|:----------------------------|:----------------------------------| |
| 152 | +|`/workorder` | provides endpoints for performing `create`, `read` and `delete` operations on work order queue. | |
| 153 | + |
| 154 | +| Operations | Description |Method| |
| 155 | +|:----------------------------|:----------------------------------|-------| |
| 156 | +| `/addOrder` | An endpoint for adding a ID to queue (enqueue). This endpoint should accept two parameters, the ID to enqueue(`requesterId`) and the time at which the ID was added to the queue(`timeOfRequest`). | `POST` | |
| 157 | +| `/getPosition/{requesterId}` | An endpoint to get the position of a specific ID in the queue. This endpoint should accept one parameter, the ID to get the position of. It should return the position of the ID in the queue indexed from 0. | `GET` | |
| 158 | +| `/getOrderList` | An endpoint for getting the list of IDs in the queue. This endpoint should return a list of IDs sorted from highest ranked to lowest. |`GET` | |
| 159 | +| `/remove/{requesterId}` | An endpoint for removing a specific ID from the queue. This endpoint should accept a single parameter, the ID to remove. |`DELETE` | |
| 160 | +|`/removeNextOrder` | An endpoint for getting the top ID from the queue and removing it (dequeue). This endpoint should return the highest ranked ID and the time it was entered into the queue. |`GET` | |
| 161 | +| `/avgWaitTime/{currentTimeInMillis}` | An endpoint to get the average wait time. This endpoint should accept a single parameter, the current time, and should return the average (mean) number of seconds that each ID has been waiting in the queue. |`GET` | |
| 162 | + |
| 163 | + |
| 164 | + |
| 165 | + |
| 166 | +---------------------------- |
| 167 | + |
| 168 | +[1]: https://github.com/spring-projects/spring-boot |
0 commit comments