|
| 1 | +# [1232. Check If It Is a Straight Line](https://leetcode.com/problems/check-if-it-is-a-straight-line/) |
| 2 | + |
| 3 | + |
| 4 | +## 题目: |
| 5 | + |
| 6 | +You are given an array `coordinates`, `coordinates[i] = [x, y]`, where `[x, y]` represents the coordinate of a point. Check if these points make a straight line in the XY plane. |
| 7 | + |
| 8 | +**Example 1**: |
| 9 | + |
| 10 | +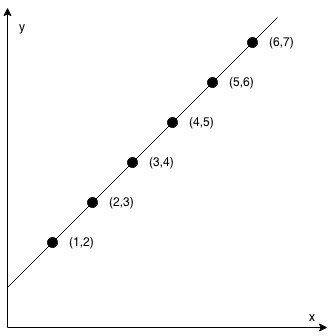 |
| 11 | + |
| 12 | + Input: coordinates = [[1,2],[2,3],[3,4],[4,5],[5,6],[6,7]] |
| 13 | + Output: true |
| 14 | + |
| 15 | +**Example 2**: |
| 16 | + |
| 17 | + |
| 18 | +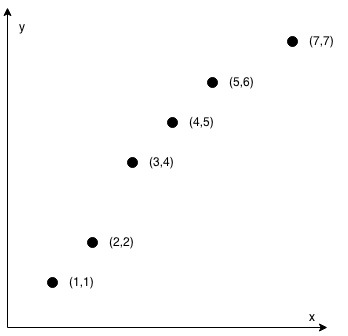 |
| 19 | + |
| 20 | + Input: coordinates = [[1,1],[2,2],[3,4],[4,5],[5,6],[7,7]] |
| 21 | + Output: false |
| 22 | + |
| 23 | +**Constraints**: |
| 24 | + |
| 25 | +- `2 <= coordinates.length <= 1000` |
| 26 | +- `coordinates[i].length == 2` |
| 27 | +- `-10^4 <= coordinates[i][0], coordinates[i][1] <= 10^4` |
| 28 | +- `coordinates` contains no duplicate point. |
| 29 | + |
| 30 | +## 题目大意 |
| 31 | + |
| 32 | + |
| 33 | +在一个 XY 坐标系中有一些点,我们用数组 coordinates 来分别记录它们的坐标,其中 coordinates[i] = [x, y] 表示横坐标为 x、纵坐标为 y 的点。 |
| 34 | + |
| 35 | +请你来判断,这些点是否在该坐标系中属于同一条直线上,是则返回 true,否则请返回 false。 |
| 36 | + |
| 37 | +提示: |
| 38 | + |
| 39 | +- 2 <= coordinates.length <= 1000 |
| 40 | +- coordinates[i].length == 2 |
| 41 | +- -10^4 <= coordinates[i][0], coordinates[i][1] <= 10^4 |
| 42 | +- coordinates 中不含重复的点 |
| 43 | + |
| 44 | + |
| 45 | + |
| 46 | +## 解题思路 |
| 47 | + |
| 48 | +- 给出一组坐标点,要求判断这些点是否在同一直线上。 |
| 49 | +- 按照几何原理,依次计算这些点的斜率是否相等即可。斜率需要做除法,这里采用一个技巧是换成乘法。例如 `a/b = c/d` 换成乘法是 `a*d = c*d` 。 |
| 50 | + |
| 51 | + |
| 52 | +## 代码 |
| 53 | + |
| 54 | +```go |
| 55 | + |
| 56 | +package leetcode |
| 57 | + |
| 58 | +func checkStraightLine(coordinates [][]int) bool { |
| 59 | + dx0 := coordinates[1][0] - coordinates[0][0] |
| 60 | + dy0 := coordinates[1][1] - coordinates[0][1] |
| 61 | + for i := 1; i < len(coordinates)-1; i++ { |
| 62 | + dx := coordinates[i+1][0] - coordinates[i][0] |
| 63 | + dy := coordinates[i+1][1] - coordinates[i][1] |
| 64 | + if dy*dx0 != dy0*dx { // check cross product |
| 65 | + return false |
| 66 | + } |
| 67 | + } |
| 68 | + return true |
| 69 | +} |
| 70 | + |
| 71 | + |
| 72 | +``` |
0 commit comments