forked from redshoga/site-cat-action
-
Notifications
You must be signed in to change notification settings - Fork 0
/
Copy pathindex.js
41 lines (36 loc) · 1.22 KB
/
index.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
const core = require("@actions/core");
const fs = require("fs");
const Octokit = require("@octokit/rest");
const isLgtmString = (text, triggerWords) => {
return text.trim().toLowerCase() === triggerWords;
};
// most @actions toolkit packages have async methods
async function run() {
try {
// action info
const repoOwner = process.env.GITHUB_REPOSITORY.split("/")[0];
const repoName = process.env.GITHUB_REPOSITORY.split("/")[1];
if (!["issue_comment"].includes(process.env.GITHUB_EVENT_NAME)) return;
// github client
const token = core.getInput("token");
const triggerWord = core.getInput("triggerWord");
const octokit = new Octokit({
auth: `token ${token}`,
});
// issue info
const issueInfo = JSON.parse(
fs.readFileSync(process.env.GITHUB_EVENT_PATH, "utf8")
);
if (!isLgtmString(issueInfo.comment.body, triggerWord)) return;
octokit.issues.createComment({
owner: repoOwner,
repo: repoName,
issue_number: issueInfo.issue.number,
body:
"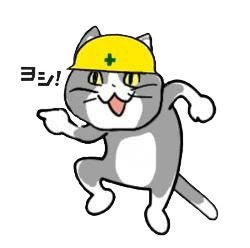",
});
} catch (error) {
core.setFailed(error.message);
}
}
await run();