forked from hedyorg/hedy
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
3335 UI idea extend skulpt with pygame4skulpt (hedyorg#3395)
Make it possible for Skulpt to execute Pygame (partially), by including PyGame4Skulpt as a external library for Skulpt. **Description** Things worthy of mentioning: 1. [PyGame4Skulpt ](https://github.com/Dustyposa/pygame4skulpt) is added to Skulpt 2. variable _hasPygame_ is used analog to the _hasTurtle_ variable, for levels that will need Pygame functionality in the future. 3. the function runPythonProgram is changed to accommodate _hasPygame_ . This way the library is only imported when necessary. 4. the function runPythonProgram is 'exported', it is accessible via the DOM. This makes it a lot easier to test programs by being able to run real Python, especially when testing Pygame related code. 5. Same canvas is being used for Turtle and Pygame code **How to test** 1. Open up any level and copy & paste the following Python code into the editor ```python import math, random width = 711 heigth = 300 print('Drawing Circles!') def get_random_color(): return random.randint(0, 255), random.randint(0, 255), random.randint(0, 255) def draw_circle(): r = d / (2 * n) kx = width / (2 * n) ky = heigth / (2 * n) canvas.fill(pygame.Color(247, 250, 252, 255)) for i in range(n): (cx, cy) = ((2 * i + 1) * kx, heigth - (2 * i + 1) * ky) pygame.draw.circle(canvas, get_random_color(), (round(cx), round(cy)), round(r), 2) pygame.display.update() d = math.sqrt(width ** 2 + heigth ** 2) n = 7 draw_circle() end = False while not end: event = pygame.event.wait() if event.type == pygame.QUIT: end = True elif event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: n += 1 draw_circle() elif event.button == 3 and n > 1: n -= 1 draw_circle() if event.type == pygame.KEYDOWN: if event.key == pygame.K_UP: n += 1 draw_circle() elif event.key == pygame.K_DOWN and n > 1: n -= 1 draw_circle() pygame.quit() ``` 2. Open up your browser console & execute the following JS ```javascript let code = hedyApp.get_trimmed_code() hedyApp.runPythonProgram(code, false, true, false, false) ``` 3. You should now be able to increase the circles with the **up arrow/right mouse click** and decrease with the **down arrow/left mouse click**, you can stop executing Pygame with the **escape button**. 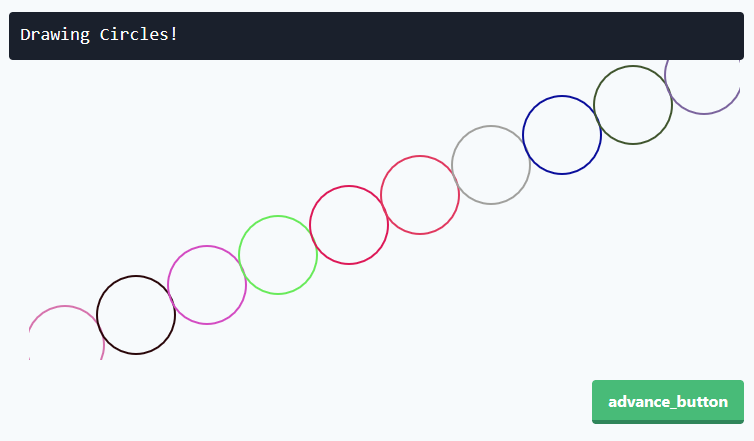
- Loading branch information
Showing
16 changed files
with
3,890 additions
and
23 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Large diffs are not rendered by default.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -68,7 +68,7 @@ interface State { | |
|
||
declare interface Window { | ||
State: State; | ||
|
||
width: int; | ||
editor?: AceAjax.Editor; | ||
} | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,52 @@ | ||
var $builtinmodule = function (name) { | ||
var mod = {}; | ||
mod.init = new Sk.builtin.func(function () { | ||
mod.__is_initialized = true; | ||
return Sk.builtin.none.none$; | ||
}); | ||
mod.quit = new Sk.builtin.func(function () { | ||
mod.__is_initialized = false; | ||
return Sk.builtin.none.none$; | ||
}); | ||
mod.get_init = new Sk.builtin.func(function () { | ||
if (mod.__is_initialized) { | ||
return Sk.ffi.remapToPy(true); | ||
} | ||
return Sk.ffi.remapToPy(false); | ||
}); | ||
mod.set_mode = new Sk.builtin.func(function (size, flags) { | ||
var f = 0; | ||
if (flags !== undefined) { | ||
f = Sk.ffi.remapToJs(flags); | ||
} | ||
if (f & PygameLib.constants.FULLSCREEN) { | ||
mod.surface = Sk.misceval.callsim(PygameLib.SurfaceType, size, true, true); | ||
} else { | ||
mod.surface = Sk.misceval.callsim(PygameLib.SurfaceType, size, false, true); | ||
} | ||
PygameLib.surface = mod.surface; | ||
return mod.surface; | ||
}); | ||
mod.get_surface = new Sk.builtin.func(function () { | ||
return PygameLib.surface; | ||
}); | ||
mod.update = new Sk.builtin.func(function () { | ||
Sk.misceval.callsim(mod.surface.update, mod.surface); | ||
}); | ||
mod.flip = new Sk.builtin.func(function () { | ||
Sk.misceval.callsim(mod.surface.update, mod.surface); | ||
}); | ||
mod.set_caption = new Sk.builtin.func(function (caption) { | ||
PygameLib.caption = Sk.ffi.remapToJs(caption); | ||
if (Sk.title_container) { | ||
Sk.title_container.innerText = PygameLib.caption; | ||
} | ||
}); | ||
mod.get_caption = new Sk.builtin.func(function () { | ||
return Sk.ffi.remapToPy(PygameLib.caption); | ||
}); | ||
mod.get_active = new Sk.builtin.func(function () { | ||
return Sk.ffi.remapToPy(document.hasFocus()); | ||
}); | ||
return mod; | ||
}; |
Oops, something went wrong.