forked from FuelLabs/sway
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
bug(fmt): do not iteratively call forc-fmt over members on the root d…
…ir (FuelLabs#4038) ## Description closes FuelLabs#4027 This is a fix for the formatter being called to format multiple times. This implements the suggested changes described in the issue, and refactors some of the code: - `format_file()` is now renamed to `write_file_formatted()`, since all it does is write to a file. - Extract logic of formatting a single file into `format_file()`. This means its reusable at 3 levels: formatting a single file, formatting a package and formatting a workspace. - Handles formatting by workspace vs package - formatting a workspace is handled slightly differently. Formatting a package has no changes - it still works the same. However, for workspaces, we want to format all files at the root, then find for each subdirectories (inclusively), all the nested directories with a manifest inside. If it exists, we are interested in formatting it. If the subdirectory happens to have a `swayfmt.toml` inside it, we will prioritize that configuration file. Generally, we should prefer member config > workspace config > default. This nuance is [explained in a comment](https://github.com/FuelLabs/sway/pull/4038/files#diff-bf9b5d7023fff817f83ae31bd01e66d2788178a0705a2c638ce79d7990cc374aR190-R193). - Extract logic of formatting a manifest into `format_manifest()` It's faster by quite a bit, with some good ol' primitive testing: 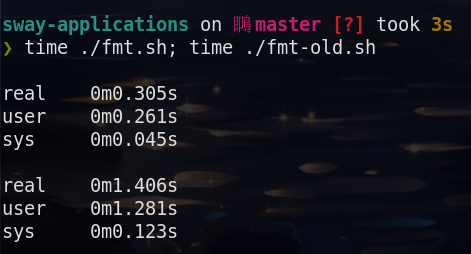 new (fmt.sh): ``` for i in `seq 1 13`; do ./forc-fmt --path AMM done ``` The above `forc-fmt` is built on `--release` and I moved it to the sway apps repo for testing. Looped it 13 times because that's the no. of projects in sway-applications. old (fmt-old.sh): ``` for i in `seq 1 13`; do forc fmt --path AMM done ``` This one is just the current formatter. ## Checklist - [x] I have linked to any relevant issues. - [x] I have commented my code, particularly in hard-to-understand areas. - [ ] I have updated the documentation where relevant (API docs, the reference, and the Sway book). - [x] I have added tests that prove my fix is effective or that my feature works. - [x] I have added (or requested a maintainer to add) the necessary `Breaking*` or `New Feature` labels where relevant. - [ ] I have done my best to ensure that my PR adheres to [the Fuel Labs Code Review Standards](https://github.com/FuelLabs/rfcs/blob/master/text/code-standards/external-contributors.md). - [x] I have requested a review from the relevant team or maintainers. --------- Co-authored-by: Sophie Dankel <[email protected]> Co-authored-by: Kaya Gökalp <[email protected]>
- Loading branch information
1 parent
1f9debf
commit 080543e
Showing
2 changed files
with
186 additions
and
69 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters