forked from pulumi/examples
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add aws-native S3 folder example (pulumi#1085)
- Loading branch information
1 parent
b2da73d
commit b14a70d
Showing
8 changed files
with
185 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,2 @@ | ||
/bin/ | ||
/node_modules/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
name: aws-native-ts-s3-folder | ||
runtime: nodejs | ||
description: A static website hosted on AWS S3 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,94 @@ | ||
[](https://app.pulumi.com/new) | ||
|
||
# Host a Static Website on Amazon S3 | ||
|
||
A static website that uses [S3's website support](https://docs.aws.amazon.com/AmazonS3/latest/dev/WebsiteHosting.html). | ||
For a detailed walkthrough of this example, see the tutorial [Static Website on AWS S3](https://www.pulumi.com/docs/tutorials/aws/s3-website/). | ||
|
||
Note: Some of the resources are not yet supported by the Native AWS provider, so we are using both the Native | ||
and Classic provider in this example. The resources will be updated to use native resources as they are | ||
available in AWS's Cloud Control API. | ||
|
||
## Deploying and running the program | ||
|
||
Note: some values in this example will be different from run to run. These values are indicated | ||
with `***`. | ||
|
||
1. Create a new stack: | ||
|
||
```bash | ||
$ pulumi stack init dev | ||
``` | ||
|
||
1. Set the AWS region: | ||
|
||
Either using an environment variable | ||
```bash | ||
$ export AWS_REGION=us-west-2 | ||
``` | ||
|
||
Or with the stack config | ||
```bash | ||
$ pulumi config set aws:region us-west-2 | ||
$ pulumi config set aws-native:region us-west-2 | ||
``` | ||
|
||
1. Restore NPM modules via `npm install` or `yarn install`. | ||
|
||
1. Run `pulumi up` to preview and deploy changes. After the preview is shown you will be | ||
prompted if you want to continue or not. | ||
|
||
```bash | ||
$ pulumi up | ||
Previewing update (dev) | ||
... | ||
Updating (dev) | ||
View Live: https://app.pulumi.com/***/aws-native-ts-s3-folder/dev/updates/1 | ||
Type Name Status | ||
+ pulumi:pulumi:Stack aws-native-ts-s3-folder-dev created | ||
+ ├─ aws-native:s3:Bucket s3-website-bucket created | ||
+ ├─ aws:s3:BucketPolicy bucketPolicy created | ||
+ ├─ aws:s3:BucketObject index.html created | ||
+ └─ aws:s3:BucketObject favicon.png created | ||
Outputs: | ||
bucketName: "***" | ||
websiteUrl: "http://***.s3-website-us-west-2.amazonaws.com" | ||
Resources: | ||
+ 5 created | ||
Duration: *** | ||
``` | ||
|
||
1. To see the resources that were created, run `pulumi stack output`: | ||
|
||
```bash | ||
$ pulumi stack output | ||
Current stack outputs (2): | ||
OUTPUT VALUE | ||
bucketName *** | ||
websiteUrl http://***.s3-website-us-west-2.amazonaws.com | ||
``` | ||
|
||
1. To see that the S3 objects exist, you can either use the AWS Console or the AWS CLI: | ||
|
||
```bash | ||
$ aws s3 ls $(pulumi stack output bucketName) | ||
2021-09-30 15:27:58 13731 favicon.png | ||
2021-09-30 15:27:58 198 index.html | ||
``` | ||
|
||
1. Open the site URL in a browser to see both the rendered HTML and the favicon: | ||
|
||
```bash | ||
$ pulumi stack output websiteUrl | ||
***.s3-website-us-west-2.amazonaws.com | ||
``` | ||
|
||
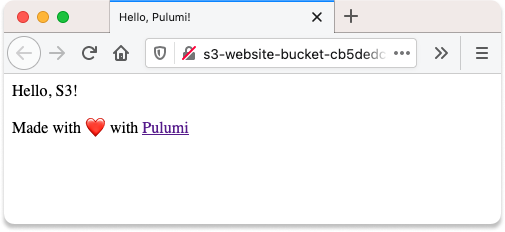 | ||
|
||
1. To clean up resources, run `pulumi destroy` and answer the confirmation question at the prompt. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
// Copyright 2016-2021, Pulumi Corporation. | ||
|
||
import * as aws from "@pulumi/aws"; | ||
import * as awsnative from "@pulumi/aws-native"; | ||
import * as pulumi from "@pulumi/pulumi"; | ||
import * as fs from 'fs'; | ||
import * as mime from "mime"; | ||
|
||
// Create a bucket and expose a website index document | ||
const siteBucket = new awsnative.s3.Bucket("s3-website-bucket", { | ||
websiteConfiguration: { | ||
indexDocument: "index.html", | ||
}, | ||
}); | ||
|
||
const siteDir = "www"; // directory for content files | ||
|
||
// For each file in the directory, create an S3 object stored in `siteBucket` | ||
for (const item of fs.readdirSync(siteDir)) { | ||
const filePath = require("path").join(siteDir, item); | ||
const siteObject = new aws.s3.BucketObject(item, { | ||
bucket: siteBucket.id, // reference the s3.Bucket object | ||
source: new pulumi.asset.FileAsset(filePath), // use FileAsset to point to a file | ||
contentType: mime.getType(filePath) || undefined, // set the MIME type of the file | ||
}); | ||
} | ||
|
||
// Set the access policy for the bucket so all objects are readable | ||
const bucketPolicy = new aws.s3.BucketPolicy("bucketPolicy", { | ||
bucket: siteBucket.id, // refer to the bucket created earlier | ||
policy: siteBucket.arn.apply(bucketArn => JSON.stringify({ | ||
Version: "2012-10-17", | ||
Statement: [{ | ||
Effect: "Allow", | ||
Principal: "*", | ||
Action: [ | ||
"s3:GetObject", | ||
], | ||
Resource: [ | ||
`${bucketArn}/*`, // | ||
], | ||
}], | ||
})), | ||
}); | ||
|
||
// Stack exports | ||
export const bucketName = siteBucket.bucketName; | ||
export const websiteUrl = siteBucket.websiteURL; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,13 @@ | ||
{ | ||
"name": "aws-native-ts-s3-bucket", | ||
"devDependencies": { | ||
"@types/node": "^8.0.0" | ||
}, | ||
"dependencies": { | ||
"@pulumi/pulumi": "^3.0.0", | ||
"@pulumi/aws": "^4.0.0", | ||
"@pulumi/aws-native": "^0.1.0", | ||
"@types/mime": "^2.0.3", | ||
"mime": "^2.5.2" | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,18 @@ | ||
{ | ||
"compilerOptions": { | ||
"strict": true, | ||
"outDir": "bin", | ||
"target": "es2016", | ||
"module": "commonjs", | ||
"moduleResolution": "node", | ||
"sourceMap": true, | ||
"experimentalDecorators": true, | ||
"pretty": true, | ||
"noFallthroughCasesInSwitch": true, | ||
"noImplicitReturns": true, | ||
"forceConsistentCasingInFileNames": true | ||
}, | ||
"files": [ | ||
"index.ts" | ||
] | ||
} |
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
<html> | ||
<head><meta charset="UTF-8"> | ||
<title>Hello, Pulumi!</title></head> | ||
<body> | ||
<p>Hello, S3!</p> | ||
<p>Made with ❤️ with <a href="https://pulumi.com">Pulumi</a></p> | ||
</body></html> |